今天的一个任务就是在移动端使用Unity下载服务器端的Zip文件并将其解压,查询了一下C#相关文档,发现C#自身库类并不能解决解压压缩文件的问题,经过查询,C#可以使用ICSharpCode.SharpZipLib.dll压缩或者解压文件夹和文件。
ICSharpCode.SharpZipLib.dll下载地址:
[官网地址](http://www.icsharpcode.net/opensource/sharpziplib/)
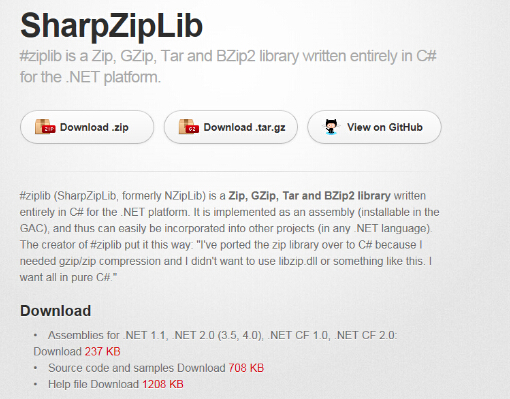
直接上代码:
/// <summary>
/// 下载
/// </summary>
/// <param name="ZipID" ZipID的名字,用于存储解压出的每一个Zip文件></param>
/// <param name="url" Zip下载地址></param>
/// <returns></returns>
IEnumerator Wait_LoadDown(string ZipID,string url)
{
WWW www = new WWW(url);
yield return www;
if (www.isDone)
{
if (www.error == null)
{
Debug.Log("下载成功");
string dir = Application.persistentDataPath;
Debug.Log(dir);
if (!Directory.Exists(dir))
Directory.CreateDirectory(dir);
yield return new WaitForEndOfFrame();
//直接使用 将byte转换为Stream,省去先保存到本地在解压的过程
XQTool.SaveZip(ZipID, url, www.bytes);
}
else
{
Debug.Log(www.error);
}
}
}
下载完成时存在立即调用解压的方法:
yield return new WaitForEndOfFrame();
//直接使用 将byte转换为Stream,省去先保存到本地在解压的过程
XQTool.SaveZip(ZipID, url, www.bytes,null);
该方法如下所示——即是解压的核心所在:
/// <summary>
/// 解压功能(下载后直接解压压缩文件到指定目录)
/// </summary>
/// <param name="wwwStream">www下载转换而来的Stream</param>
/// <param name="zipedFolder">指定解压目标目录(每一个Obj对应一个Folder)</param>
/// <param name="password">密码</param>
/// <returns>解压结果</returns>
public static bool SaveZip(string ZipID,string url,byte[] ZipByte, string password)
{
bool result = true;
FileStream fs = null;
ZipInputStream zipStream = null;
ZipEntry ent = null;
string fileName;
ZipID = Application.persistentDataPath + "/" + ZipID;
Debug.Log(ZipID);
if (!Directory.Exists(ZipID))
{
Directory.CreateDirectory(ZipID);
}
try
{
//直接使用 将byte转换为Stream,省去先保存到本地在解压的过程
Stream stream = new MemoryStream(ZipByte);
zipStream = new ZipInputStream(stream);
if (!string.IsNullOrEmpty(password))
{
zipStream.Password = password;
}
while ((ent = zipStream.GetNextEntry()) != null)
{
if (!string.IsNullOrEmpty(ent.Name))
{
fileName = Path.Combine(ZipID, ent.Name);
#region Android
fileName = fileName.Replace('\\', '/');
if (fileName.EndsWith("/"))
{
Directory.CreateDirectory(fileName);
continue;
}
#endregion
fs = File.Create(fileName);
int size = 2048;
byte[] data = new byte[size];
while (true)
{
size = zipStream.Read(data, 0, data.Length);
if (size > 0)
{
//fs.Write(data, 0, data.Length);
fs.Write(data, 0, size);//解决读取不完整情况
}
else
break;
}
}
}
}
catch (Exception e)
{
Debug.Log(e.ToString());
result = false;
}
finally
{
if (fs != null)
{
fs.Close();
fs.Dispose();
}
if (zipStream != null)
{
zipStream.Close();
zipStream.Dispose();
}
if (ent != null)
{
ent = null;
}
GC.Collect();
GC.Collect(1);
}
return result;
}